It's important to create test frameworks for your programs. In Java we use the jUnit system to do so. Here is an example of setting up a unit tester to make sure that that a method returns the values it's supposed to.
First, let's look at the method. I'm using it to convert values that are bounded from 0 to 1023 into values from 0 to 100. I do this before graphing the results. The class is called GraphPeriodicUpdate. The method is called normalizeValue. It takes an integer and returns an integer:
// Conversion of sensor values from 0 to 1023 into 0 to 100;
static public int normalizeYValue(int originalValue){
final float MAXVALUE = 1023.0F;
final float MINVALUE = 0.0F;
final int ERRORVALUE = -1000;
int theReturnValue;
if((originalValue > MAXVALUE)){
theReturnValue = ERRORVALUE;
}
else if ( (originalValue < MINVALUE) ){
theReturnValue = ERRORVALUE;
}
else{
theReturnValue = (int)(100.0*((float)(originalValue)*((1.0)/(MAXVALUE-MINVALUE))));
}
return theReturnValue;
}
The method is designed to return -1000 if the inputs are outside of the 0 to 1023 boundaries. Now, my unit tester should ensure that
- the method returns the -1000 error value when faced with inputs that are less than 0 or greater than 1023, and
- the method only returns values from 0 to 100 if the inputs are 0 to 1023.
Here's how I can do that:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertTrue;
import java.util.Random;
public class theUnitTest {
final int MAXINPUT = 1023;
final int MININPUT = 0;
final int ERRORVALUE = -1000;
final int MINRESULT = 0;
final int MAXRESULT = 100;
@Test
public void testTheGraphNormalizingMethod(){
// test to make sure that -1000 is returned for values above 1023 ...
System.out.println("Testing against too positive input...that it returns an error value of " + ERRORVALUE);
String theErrorMessage0 = "Error: wrong value returned for an input above 1023.";
assertEquals(theErrorMessage0, ERRORVALUE,GraphPeriodicUpdate.normalizeYValue(MAXINPUT+1));
assertEquals(theErrorMessage0, ERRORVALUE,GraphPeriodicUpdate.normalizeYValue(MAXINPUT*1000));
// ... or below 0.
System.out.println("Testing against too negative input... that it returns an error value of " + ERRORVALUE);
String theErrorMessage1 = "Error: wrong value returned for an input below 0.";
assertEquals(theErrorMessage1, ERRORVALUE,GraphPeriodicUpdate.normalizeYValue(MININPUT-1));
assertEquals(theErrorMessage1, ERRORVALUE,GraphPeriodicUpdate.normalizeYValue((MININPUT-1)*1000));
// TESt to see how it behaves for values from 0 to 1023
// if there's a problem, print a message saying so.
System.out.println("Testing against values from " + MININPUT + " to " + MAXINPUT);
int theResult;
theResult = GraphPeriodicUpdate.normalizeYValue(MININPUT);
String theErrorMessage2 = "Error: The result shouldn't be below 0"; // show this if the test fails.
assertTrue(theErrorMessage2, theResult >= MINRESULT);
theResult = GraphPeriodicUpdate.normalizeYValue(MAXINPUT+1);
String theErrorMessage3 = "Error: The result shouldn't be above 100"; // show this if the test fails.
assertTrue(theErrorMessage3, theResult <= MAXRESULT);
// Throw at it a hundred random numbers, bounded from min to max values, to see if it fails...
for (int i = 0; i < 100; i++) {
System.out.print(".");
Random randomObject = new Random();
int randValue = randomObject.nextInt(MAXINPUT+1);
theResult = GraphPeriodicUpdate.normalizeYValue(randValue);
String theErrorMessage4 ="Error: The result is _not_ between 0 and 100";
assertTrue(theErrorMessage4, ((theResult <= MAXRESULT)&&(theResult>=MINRESULT)));
}
}
}
I've made this unit tester a lot more verbose than it has to be. To run the unit test, go into the file that you have defined the unit test class in and press the "run" icon:

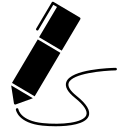
James Andrew Smith is a Professional Engineer and Associate Professor in the Electrical Engineering and Computer Science Department of York University's Lassonde School, with degrees in Electrical and Mechanical Engineering from the University of Alberta and McGill University. Previously a program director in biomedical engineering, his research background spans robotics, locomotion, human birth and engineering education. While on sabbatical in 2018-19 with his wife and kids he lived in Strasbourg, France and he taught at the INSA Strasbourg and Hochschule Karlsruhe and wrote about his personal and professional perspectives. James is a proponent of using social media to advocate for justice, equity, diversity and inclusion as well as evidence-based applications of research in the public sphere. You can find him on Twitter. Originally from Québec City, he now lives in Toronto, Canada.