Firmata is a set of Arduino projects that are aimed at helping solve a particular class of problems with embedded devices: having a main computer send and receive data from an Arduino, typically in a simple control or data-logging application. This is similar to how people use the Arduino support for Matlab.
In Java we use Firmata4j, one of three Java implementations on the host computer side.
Here is a preview video:
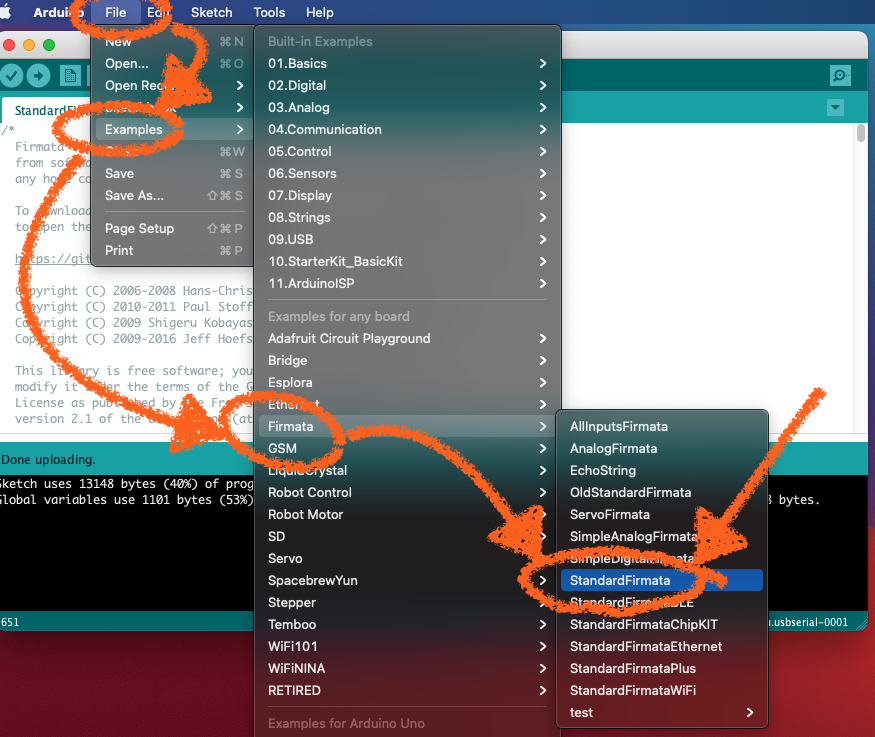
Arduino IDE
Using the Arduino IDE, load the StandardFirmata on to your Arduino board:
- Select the board (e.g. Tools -> Board -> Arduino UNO)
- Select the port (e.g. Tools -> Port -> /dev/cu.usbserial-0001 or COM3, etc.)
- Select the firmware (File -> Examples -> Firmata -> StandardFirmata)
- Compile and download StandardFirmata to your Arduino-compatible board.
Now, open up the Serial Monitor window in the Arduino IDE, set it to 57,600 baud and you should see a message appear that says "StandardFirmata.ino". If you don't, check that you're at 57600 baud on your monitor and then hit the reset button on the Arduino board.
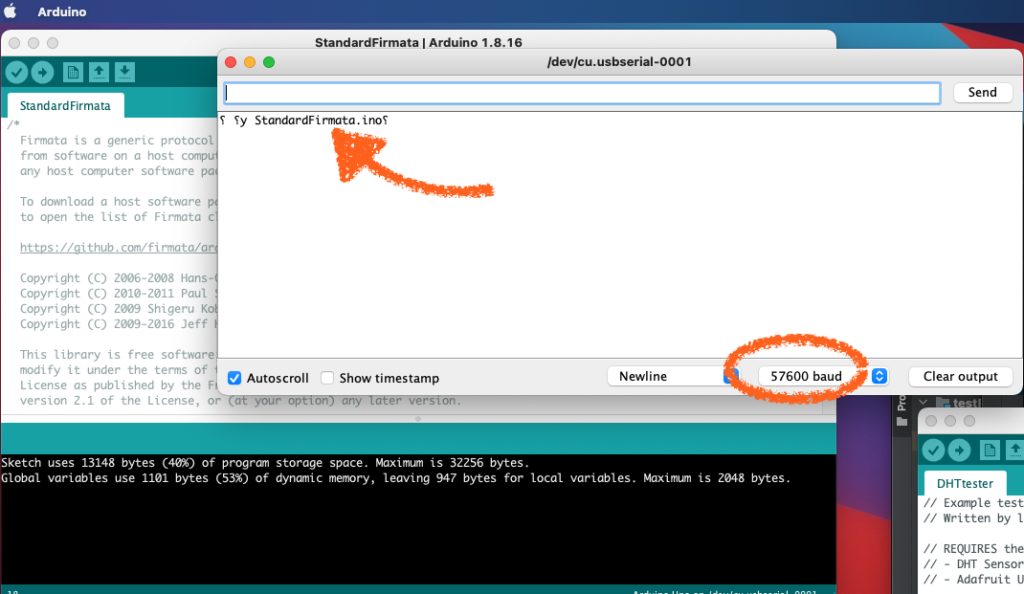
You can also connect to the board using CoolTerm or RealTerm. These terminal programs offer more detailed ways of looking at the serial data.
Java program
In order to do useful work you'll need to write a client program to interact with your board. It's 2022, so we'll use the latest version of IntelliJ (2021.3 CE) and the JDK (17).
Start a new project in IntelliJ and select JDK 17 (e.g. Oracle OpenJDK 17.0.1). I'll call my project FirmataTest8 but you can call it anything else you want.
Add a new Java Class in your project. I'll call mine firmataTest8. Inside this project, add two Java classes: Pins.java and FirmataTestClass.java.
Here is FirmataTestClass.java:
// Original source by Richard Robinson, Dec 2021.
// Updated by James Andrew Smith, Dec 2021.
import org.firmata4j.firmata.FirmataDevice;
import java.io.IOException;
public class firmataTestClass {
public static void main(String[] args) throws IOException, InterruptedException {
var device = new FirmataDevice("/dev/cu.usbserial-0001"); // Change to your serial port
device.start();
device.ensureInitializationIsDone();
// LED assumed to be on D4
// Change in Pins if you have a different pin.
var led = device.getPin(Pins.D4);
// infinite loop to send LED on/off signal to Arduino with LED on D4
while(true) {
led.setValue(0);
Thread.sleep(500);
led.setValue(1);
Thread.sleep(500);
}
}
}
And here is the Pins.java class:
/**
* Maps the Arduino pin labels to those of Firmata
* Original by Richard Robinson, Dec. 2021.
*/
public class Pins {
static final int D4 = 4; // LED
private Pins() {
}
}
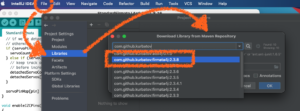
Import two packages with Maven
Next, you need to import two packages: firmata4j and slf4j. Start with firmata4j:
File -> Project Structure -> Libraries -> + -> From Maven
Search for com.github.kurbatov and choose firmata4j:2.3.8 (e.g. com.github.kurbatov:firmata4j:2.3.8
)
Next, you likely need to also add in slf4j explicitly into your project, otherwise you may get a "class path" error at run time. To do this, use Maven again:
File -> Project Structure -> Libraries -> + -> From Maven
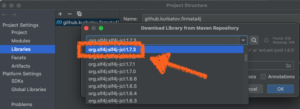
and search for org.slf4j and then choose slf4j-jcl:1.7.3. Be careful. There are lots of options here. You want the "JCL" library and you want to choose the 1.7.3 version of it (e.g. org.slf4j:slf4j-jcl:1.7.3
).
When it's all done you should see that there are two libraries downloaded and assocaited with your Java project.
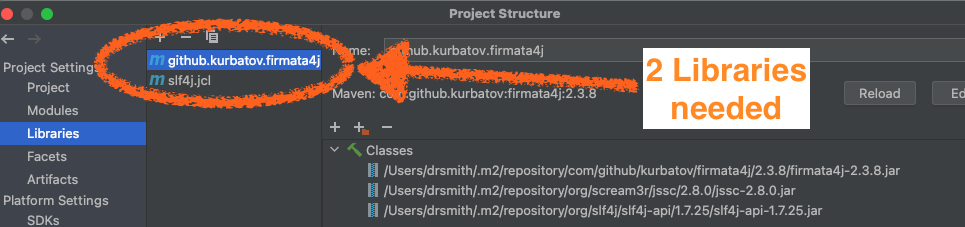
Next, compile and run. You should see the D4 LED blinking.
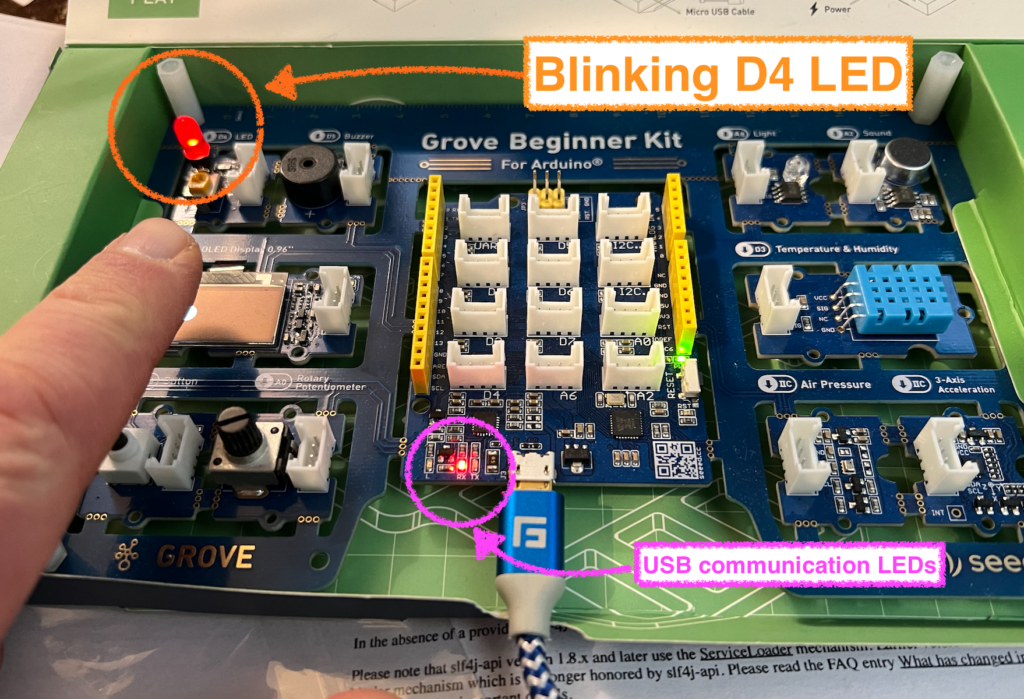
Notes on versions
As of Arduino 1.8.16 the Firmata version is 2.5. You will see a warning:
WARNING: Current version of firmata protocol on device (2.5) differs from version supported by firmata4j (2.3).
Don't downgrade to 2.3. It's not worth it. You'll get errors about i2c support. So stick to 2.5.

Conclusion
That's it. There is more you can do, but this will allow you to make sure that you've got communicaiton between your Arduino board and your Java program. For those of you using a different board than the Grove Beginner Kit for Arduino, you'll need to choose a different LED, like D13.
Many thanks to Richard Robinson for his work on the original demo!
Telemetrix update to StandardFirmata
A very recent addition to the Firmata family is Alan Yorkink's Telemetrix. This appears to provide more hardware support than Standard Firmata. We'll have to test it out in the future. But, for the time being, we'll stick to Standard Firmata.
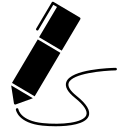
James Andrew Smith is a Professional Engineer and Associate Professor in the Electrical Engineering and Computer Science Department of York University's Lassonde School, with degrees in Electrical and Mechanical Engineering from the University of Alberta and McGill University. Previously a program director in biomedical engineering, his research background spans robotics, locomotion, human birth and engineering education. While on sabbatical in 2018-19 with his wife and kids he lived in Strasbourg, France and he taught at the INSA Strasbourg and Hochschule Karlsruhe and wrote about his personal and professional perspectives. James is a proponent of using social media to advocate for justice, equity, diversity and inclusion as well as evidence-based applications of research in the public sphere. You can find him on Twitter. Originally from Québec City, he now lives in Toronto, Canada.