As part of a research project I'm resurrecting some KL25Z boards and ChipKit basic I/O shield boards and seeing if they can be used together. The ChipKit has some pushbuttons and LEDs. The following code, with the help of single-stepping and the debugger can get the LED bank and BTN1 on the ChipKit I/O board to work. The BTN1 is working on polling only.
/**
* @file KL25Z_ChipKit_ButtonLED_test.c
* @brief Application entry point.
*/
#include <stdio.h>
#include "board.h"
#include "peripherals.h"
#include "pin_mux.h"
#include "clock_config.h"
#include "MKL25Z4.h"
#include "fsl_debug_console.h"
/* TODO: insert other include files here. */
/* TODO: insert other definitions and declarations here. */
void led_init_chipkit();
void switch_init_chipkit();
void button_init_chipkit();
/*
* @brief Application entry point.
*/
int main(void) {
/* Init board hardware. */
BOARD_InitBootPins();
BOARD_InitBootClocks();
BOARD_InitBootPeripherals();
#ifndef BOARD_INIT_DEBUG_CONSOLE_PERIPHERAL
/* Init FSL debug console. */
BOARD_InitDebugConsole();
#endif
PRINTF("Hello World\r\n");
led_init_chipkit();
switch_init_chipkit();
button_init_chipkit();
/* Force the counter to be placed into memory. */
volatile static int i = 0 ;
/* Enter an infinite loop, just incrementing a counter. */
while(1) {
i++ ;
/* 'Dummy' NOP to allow source level single stepping of
tight while() loop */
__asm volatile ("nop");
}
return 0 ;
}
/* ----------------------------------------
*
* PTC7, 0,3,4,5,6,10,11
* ---------------------------------------- */
void led_init_chipkit()
{
__disable_irq(); /* disable all IRQs */
SIM->SCGC5 |= SIM_SCGC5_PORTC_MASK; /* enable clock to Port C */
PORTC->PCR[0] = 0x100; /* PTC0 pin as GPIO via mux */
PORTC->PCR[3] = 0x100; /* PTC3 pin as GPIO */
PORTC->PCR[4] = 0x100; /* PTC4 pin as GPIO */
PORTC->PCR[5] = 0x100; /* PTC5 pin as GPIO */
PORTC->PCR[6] = 0x100; /* PTC6 pin as GPIO */
PORTC->PCR[10] = 0x100; /* PTC10 pin as GPIO */
PORTC->PCR[11] = 0x100; /* PTC11 pin as GPIO */
PTC->PDDR |= (1UL<<0)|(1UL<<3)|(1UL<<4)|(1UL<<5)|(1UL<<6)|(1UL<<10)|(1UL<<11); // bits 0,3,4,5,6,10,11 set to 1
//PTB->PDOR |= (1UL<<0)|(1UL<<3)|(1UL<<4)|(1UL<<5)|(1UL<<6)|(1UL<<10)|(1UL<<11);
PTC->PSOR |= (1UL<<0)|(1UL<<3)|(1UL<<4)|(1UL<<5)|(1UL<<6)|(1UL<<10)|(1UL<<11); // Set (LEDs on)
PTC->PCOR |= (1UL<<0)|(1UL<<3)|(1UL<<4)|(1UL<<5)|(1UL<<6)|(1UL<<10)|(1UL<<11); // Clear
__enable_irq(); /* global enable IRQs */
}
/*
* PTD4
*
*/
void switch_init_chipkit()
{
volatile uint32_t myvalue = 0;
__disable_irq(); /* disable all IRQs */
SIM->SCGC5 |= SIM_SCGC5_PORTD_MASK; /* enable clock to Port D */
PORTD->PCR[4] = 0x100; /* PTD4 pin as GPIO via mux*/
PTD->PDDR &= ~(1UL<<4); // Bit 4 set to 0.
myvalue = (PTD->PDIR >> 4) & 0x1; /* read one bit (bit 4) */
myvalue = (PTD->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTD->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTD->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTD->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTD->PDIR >> 4) & 0x1; /* read one bit*/
__enable_irq(); /* global enable IRQs */
}
/*
* PTA4
*
*/
void button_init_chipkit()
{
volatile uint32_t myvalue = 0;
__disable_irq(); /* disable all IRQs */
SIM->SCGC5 |= SIM_SCGC5_PORTA_MASK; /* enable clock to Port A */
PORTA->PCR[4] = 0x100; /* PTA4 pin as GPIO via mux*/
PTA->PDDR &= ~(1UL<<4); // Bit 4 set to 0.
myvalue = (PTA->PDIR >> 4) & 0x1; /* read one bit (bit 4) */
myvalue = (PTA->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTA->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTA->PDIR >> 4) & 0x1; /* read one bit*/
myvalue = (PTA->PDIR >> 4) & 0x1; /* read one bit*/
` myvalue = (PTA->PDIR >> 4) & 0x1; /* read one bit*/
__enable_irq(); /* global enable IRQs */
}
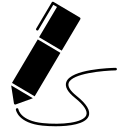
James Andrew Smith is a Professional Engineer and Associate Professor in the Electrical Engineering and Computer Science Department of York University's Lassonde School, with degrees in Electrical and Mechanical Engineering from the University of Alberta and McGill University. Previously a program director in biomedical engineering, his research background spans robotics, locomotion, human birth and engineering education. While on sabbatical in 2018-19 with his wife and kids he lived in Strasbourg, France and he taught at the INSA Strasbourg and Hochschule Karlsruhe and wrote about his personal and professional perspectives. James is a proponent of using social media to advocate for justice, equity, diversity and inclusion as well as evidence-based applications of research in the public sphere. You can find him on Twitter. Originally from Québec City, he now lives in Toronto, Canada.